Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
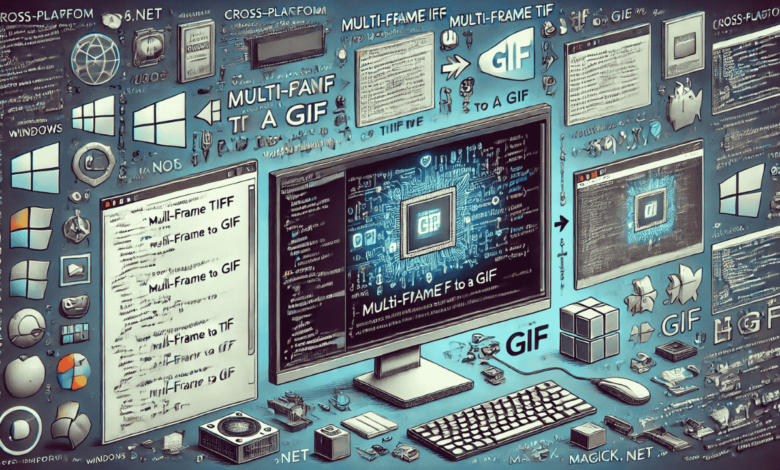
In image processing and digital workflows, converting multi-frame TIFF files to GIF format is a common requirement. Whether you’re building software for data visualization, animations, or web content, understanding this conversion process is crucial. For developers working within cross-platform .NET environments, this task demands a careful selection of tools and techniques to ensure compatibility and efficiency.
This comprehensive guide covers everything you need to know about converting multi-frame TIFF images to GIFs in cross-platform .NET applications.
Understanding Multi-Frame TIFF and GIF Formats
Before diving into the conversion process, let’s take a closer look at these file formats:
Multi-Frame TIFF
- Tagged Image File Format (TIFF): Known for its versatility, TIFF supports multiple images (frames) within a single file. It’s commonly used in professional photography, medical imaging, and geospatial applications because it stores high-quality photos.
- Multi-Frame Capability: A single TIFF file can contain multiple frames, making it ideal for storing image sequences, layered graphics, or animations.
- Advantages: Lossless compression, high resolution, and support for multiple color spaces.
GIF
- Graphics Interchange Format (GIF): A popular image format known for supporting animations. GIFs are widely used online due to their small file size and universal compatibility.
- Animation Support: A GIF file can display multiple frames in sequence, creating an animated effect.
- Advantages: Lightweight, supported by all major browsers and platforms.
Challenges in Cross-Platform .NET Environments
.NET provides a robust ecosystem for building cross-platform applications. However, some challenges arise when handling complex image processing tasks like TIFF-to-GIF conversion:
- Library Compatibility: Not all .NET libraries support cross-platform functionality. For example, System.Drawing.Common is primarily optimized for Windows.
- Performance: Processing large TIFF files with multiple frames can be resource-intensive.
- Error Handling: TIFF files may vary in structure, requiring robust error handling during conversion.
Recommended Libraries for Cross-Platform Conversion
To effectively handle TIFF-to-GIF conversion in a cross-platform .NET environment, consider using the following libraries:
ImageSharp
- Overview: An open-source, cross-platform 2D graphics and image processing library in .NET.
- Key Features:
- Supports a wide variety of formats, including TIFF and GIF.
- Provides an intuitive API for loading, manipulating, and saving images.
- Cross-platform compatibility (Windows, Linux, macOS).
Magick.NET
- Overview: A .NET wrapper for the powerful ImageMagick library.
- Key Features:
- Advanced image manipulation capabilities.
- Support for various formats, including multi-frame TIFF and animated GIF.
- High performance and extensive documentation.
SkiaSharp
- Overview: A cross-platform 2D graphics library based on Google’s Skia.
- Key Features:
- Suitable for advanced graphics rendering.
- Supports various image formats and cross-platform environments.
Step-by-Step Guide: Converting Multi-Frame TIFF to GIF
Follow these steps to convert multi-frame TIFF files to GIF format using .NET:
Step 1: Set Up Your .NET Environment
Ensure your development environment is ready:
- Install the latest .NET SDK.
- Create a new .NET project:
- dotnet new console -n TiffToGifConverter
- cd TiffToGifConverter
- Add the required library (e.g., ImageSharp):
- Dotnet added the package SixLabors.ImageSharp
Step 2: Load the Multi-Frame TIFF
Use the library to load the TIFF file. For example, using ImageSharp:
Using SixLabors.ImageSharp;
using SixLabors.ImageSharp.PixelFormats;
using SixLabors.ImageSharp.Formats.Tiff;
string tiffFilePath = “path/to/multiframe.tiff”;
using Image<Rgba32> tiffImage = Image.Load<Rgba32>(tiffFilePath);
Step 3: Process Frames and Create GIF
Iterate through the TIFF frames and save them as an animated GIF:
using SixLabors.ImageSharp.Formats.Gif;
string gifFilePath = “path/to/output.gif”;
var gifEncoder = new GifEncoder();
using (var gif = new Image<Rgba32>(tiffImage.Width, tiffImage.Height))
{
for each (var frame in tiff image.Frames)
{
gif.Frames.AddFrame(frame.Clone());
}
gif.Save(gifFilePath, gifEncoder);
}
Step 4: Test Across Platforms
- Deploy and test your application on Windows, Linux, and macOS.
- Verify that the GIF output meets your requirements on all platforms.
Optimization Tips
To improve performance and output quality:
Compression Settings
- Optimize GIF compression to reduce file size while maintaining visual quality.
- Use libraries that support advanced compression techniques.
Frame Timing
- Adjust frame delays to create smooth animations. This is particularly important for GIFs intended for web use.
Error Handling
- Handle unsupported formats or corrupted TIFF files gracefully to avoid crashes.
Resource Management
- Properly dispose of image objects to prevent memory leaks, especially when working with large TIFF files.
Use Cases and Applications
Converting multi-frame TIFF files to GIFs has a wide range of applications:
- Web Animations: Create lightweight, animated visuals for websites.
- Data Visualization: Convert scientific or medical imaging sequences into shareable GIFs.
- Social Media: Prepare animations for platforms that prefer GIF format.
Conclusion
Converting multi-frame TIFF images to GIF format in cross-platform .NET environments is a valuable skill for developers. You can efficiently handle this task by leveraging powerful libraries like ImageSharp, Magick.NET, and SkiaSharp while ensuring compatibility across different platforms. Following best optimization and error-handling practices will provide smooth performance and high-quality results.
FAQs: Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
What is a Multi-Frame TIFF file?
A Multi-Frame TIFF (Tagged Image File Format) is a TIFF file containing multiple images or frames within a single file. This makes it suitable for storing image sequences, animations, or layered graphics. It is often used in professional fields like medical imaging, photography, and geospatial applications due to its high-quality lossless compression.
What is the GIF format, and how does it differ from TIFF?
GIF (Graphics Interchange Format) is an image format that supports animations by displaying multiple frames in sequence. Unlike TIFF, mainly used for high-quality static images, GIFs are lightweight and widely supported by browsers and platforms, making them ideal for web animations and social media.
Why convert Multi-Frame TIFF to GIF?
Converting Multi-Frame TIFF to GIF is often done to create lightweight, animated images for use on websites, social media platforms, or data visualization. GIFs are easier to share and view across different devices and platforms due to their smaller file size and broad compatibility.
Can I convert Multi-Frame TIFF to GIF on Linux or macOS?
Yes, using libraries like ImageSharp and Magick.NET, you can convert Multi-Frame TIFF to GIF on Windows, Linux, and macOS. These libraries are cross-platform, ensuring compatibility across all major operating systems.
What if the TIFF file contains corrupted or unsupported data?
In cases of corrupted or unsupported data, implement robust error handling in your code to gracefully manage these errors, ensuring the application doesn’t crash and providing informative feedback to the user.